Build CRUD Application with jQuery EasyUI
Tutorial » Build CRUD Application with jQuery EasyUI
It has become a common necessily for web application to collect data and manage it properly. CRUD allows us to generate pages to list and edit database records. This tutorial will show you how to implement a CRUD DataGrid using jQuery EasyUI framework.
We will use following plugins:
- datagrid: show the user list data.
- dialog: create or edit a single user information.
- form: used to submit form data.
- messager: to show some operation messages.
Step 1: Prepare database
We will use MySql database to store user information. Create database and 'users' table.
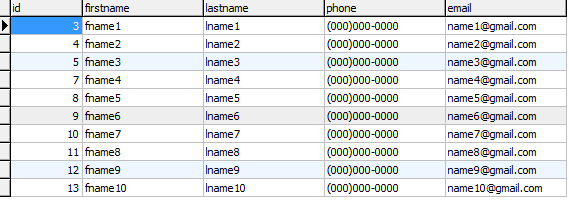
Step 2: Create DataGrid to display user information
Create the DataGrid with no javascript code.
- <table id="dg" title="My Users" class="easyui-datagrid" style="width:550px;height:250px"
- url="get_users.php"
- toolbar="#toolbar"
- rownumbers="true" fitColumns="true" singleSelect="true">
- <thead>
- <tr>
- <th field="firstname" width="50">First Name</th>
- <th field="lastname" width="50">Last Name</th>
- <th field="phone" width="50">Phone</th>
- <th field="email" width="50">Email</th>
- </tr>
- </thead>
- </table>
- <div id="toolbar">
- <a href="#" class="easyui-linkbutton" iconCls="icon-add" plain="true" onclick="newUser()">New User</a>
- <a href="#" class="easyui-linkbutton" iconCls="icon-edit" plain="true" onclick="editUser()">Edit User</a>
- <a href="#" class="easyui-linkbutton" iconCls="icon-remove" plain="true" onclick="removeUser()">Remove User</a>
- </div>
We don't need to write any javascript code and we can show the user list as following image:
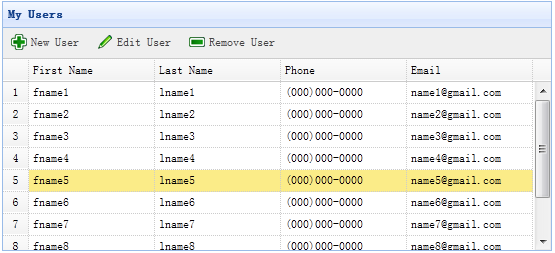
The DataGrid use the 'url' property that is assigned to 'get_users.php' to retrieve data from server.
Code of get_users.php file
- $rs = mysql_query('select * from users');
- $result = array();
- while($row = mysql_fetch_object($rs)){
- array_push($result, $row);
- }
- echo json_encode($result);
Step 3: Create form dialog
To create or edit a user, we use the same dialog.
- <div id="dlg" class="easyui-dialog" style="width:400px;height:280px;padding:10px 20px"
- closed="true" buttons="#dlg-buttons">
- <div class="ftitle">User Information</div>
- <form id="fm" method="post">
- <div class="fitem">
- <label>First Name:</label>
- <input name="firstname" class="easyui-validatebox" required="true">
- </div>
- <div class="fitem">
- <label>Last Name:</label>
- <input name="lastname" class="easyui-validatebox" required="true">
- </div>
- <div class="fitem">
- <label>Phone:</label>
- <input name="phone">
- </div>
- <div class="fitem">
- <label>Email:</label>
- <input name="email" class="easyui-validatebox" validType="email">
- </div>
- </form>
- </div>
- <div id="dlg-buttons">
- <a href="#" class="easyui-linkbutton" iconCls="icon-ok" onclick="saveUser()">Save</a>
- <a href="#" class="easyui-linkbutton" iconCls="icon-cancel" onclick="javascript:$('#dlg').dialog('close')">Cancel</a>
- </div>
The dialog is created with no javascript code also.
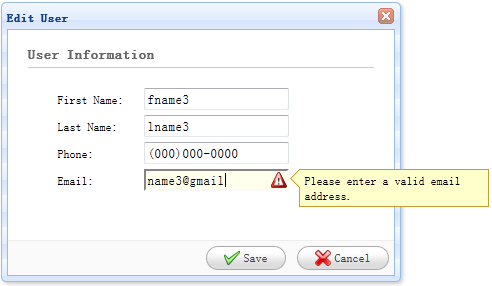
Step 4: Implement creating and editing a user
When create a user, we open the dialog and clear form data.
- function newUser(){
- $('#dlg').dialog('open').dialog('setTitle','New User');
- $('#fm').form('clear');
- url = 'save_user.php';
- }
When edit a user, we open the dialog and load form data from the selected datagrid row.
- var row = $('#dg').datagrid('getSelected');
- if (row){
- $('#dlg').dialog('open').dialog('setTitle','Edit User');
- $('#fm').form('load',row);
- url = 'update_user.php?id='+row.id;
- }
The 'url' stores the URL address where the form will post to when save the user data.
Step 5: Save the user data
To save the user data we use the following code:
- function saveUser(){
- $('#fm').form('submit',{
- url: url,
- onSubmit: function(){
- return $(this).form('validate');
- },
- success: function(result){
- var result = eval('('+result+')');
- if (result.success){
- $('#dlg').dialog('close'); // close the dialog
- $('#dg').datagrid('reload'); // reload the user data
- } else {
- $.messager.show({
- title: 'Error',
- msg: result.msg
- });
- }
- }
- });
- }
Before submit the form, the 'onSubmit' function will be called, in which we can validate the form field value. When the form field values are submited successfully, close the dialog and reload the datagrid data.
Step 6: Remove a user
To remove a user, we use the following code:
- function removeUser(){
- var row = $('#dg').datagrid('getSelected');
- if (row){
- $.messager.confirm('Confirm','Are you sure you want to remove this user?',function(r){
- if (r){
- $.post('remove_user.php',{id:row.id},function(result){
- if (result.success){
- $('#dg').datagrid('reload'); // reload the user data
- } else {
- $.messager.show({ // show error message
- title: 'Error',
- msg: result.msg
- });
- }
- },'json');
- }
- });
- }
- }
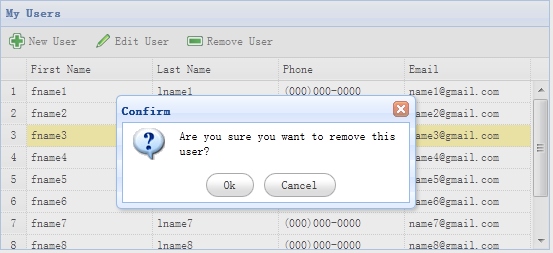
Before remove a row, we will display a confirm dialog to let user to determine whether to really remove the row data. When remove data successfully, call 'reload' method to refresh the datagrid data.
Step 7: Run the Code
Run the code in your browser with MySQL started.
So, you now know the basics of CRUD in jQuery EasyUI framework. Press below link to start the demo application.
Download the EasyUI example:
'J-Query' 카테고리의 다른 글
jQuey eq form 값 반디 모음 1 (0) | 2012.06.22 |
---|---|
select 태그에서 선택된 option 값 알아보기 (1) | 2012.06.15 |
슬라이드 시계 (0) | 2012.06.13 |
JQuery 시계 (0) | 2012.06.13 |
조작하려는 엘리먼트 선택하기 (0) | 2012.06.07 |